Recently I had an opportunity to work with Django REST framework on Kraken’s new product - kWiki (a project documentation platform that is easy to use and quick to integrate). It didn’t take too long to see the benefits of its built-in support for documenting APIs. It automatically generates documentation for your API endpoints and all you need to do to enable this ‘cool’ feature is include it in your URL configuration file. For more details please check Django REST framework - Documenting your API.
However, if you would prefer to document your API using other tools, Django REST framework has a support for a couple of them. One of them is Django REST Swagger, used for generating well known Swagger documentation. We’ll quickly go over a few steps necessary to get it up and running:
Prerequisites: Python 2.7, 3.5 or 3.6, Django 1.8+, Django REST framework 3.5.1+
- Install django-rest-swagger package:
pip install django-rest-swagger
. - Add
rest_framework_swagger
toINSTALLED_APPS
inside your settings:
INSTALLED_APPS = [
...
'rest_framework_swagger',
...
]
- For a quick start, use shortcut method
get_swagger_view
which will generate Swagger UI documentation for your API. This method have two optional parameters title (of your Swagger documentation) and url (to your Swagger documentation). You need to add to your URL configuration the following code:
...
from rest_framework.documentation import include_docs_urls
...
schema_view = get_swagger_view(title="Swagger Docs")
url patterns = [
...
url(r'^docs/', schema_view),
...
]
And that’s all you need to have!
In order to show you a live example, I created a simple dogs application along with a Dog
model, DogSerializer
and DogViewSet
so we can test it out.
...
class DogSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = Dog
fields = ('name', 'age')
...
As you will see in the following example, you can also add a short description for your API. Just make sure you are using the appropriate method names on the ModelViewSet()
.
...
class DogViewSet(viewsets.ModelViewSet):
"""
retrieve:
Return the given dog.
list:
Return a list of all dogs.
create:
Create a new dog.
destroy:
Delete a dog.
update:
Update a dog.
partial_update:
Update a dog.
"""
queryset = Dog.objects.all()
serializer_class = DogSerializer
...
If you run the server and go to http://localhost:8000/docs/
, you should be able to see the following:
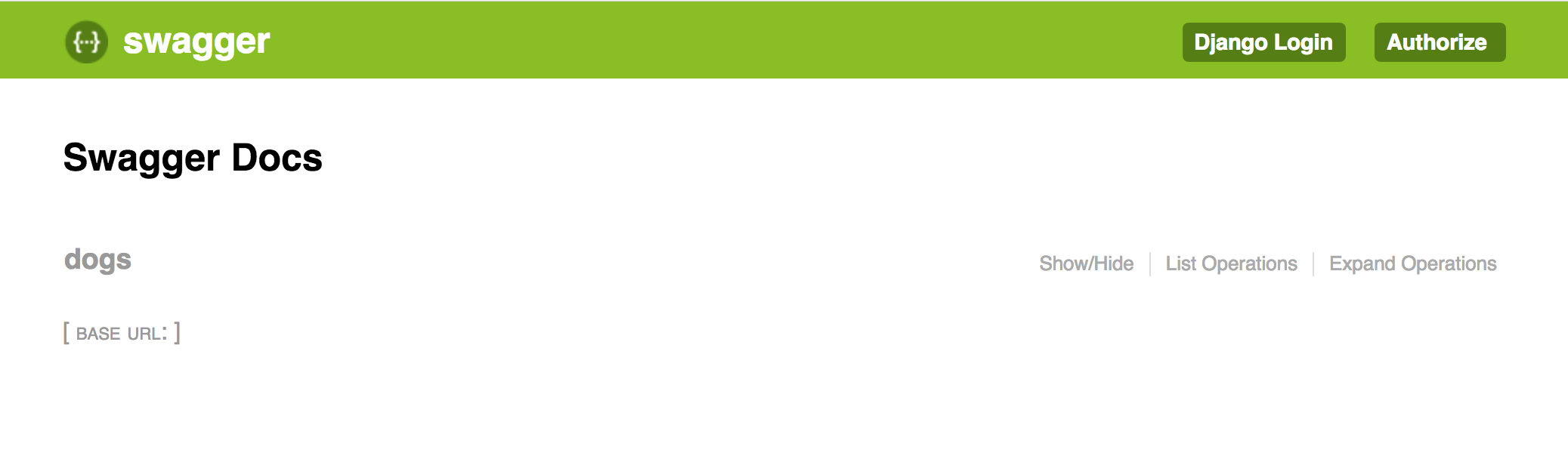
Under “dogs” are all available endpoints along with their descriptions:
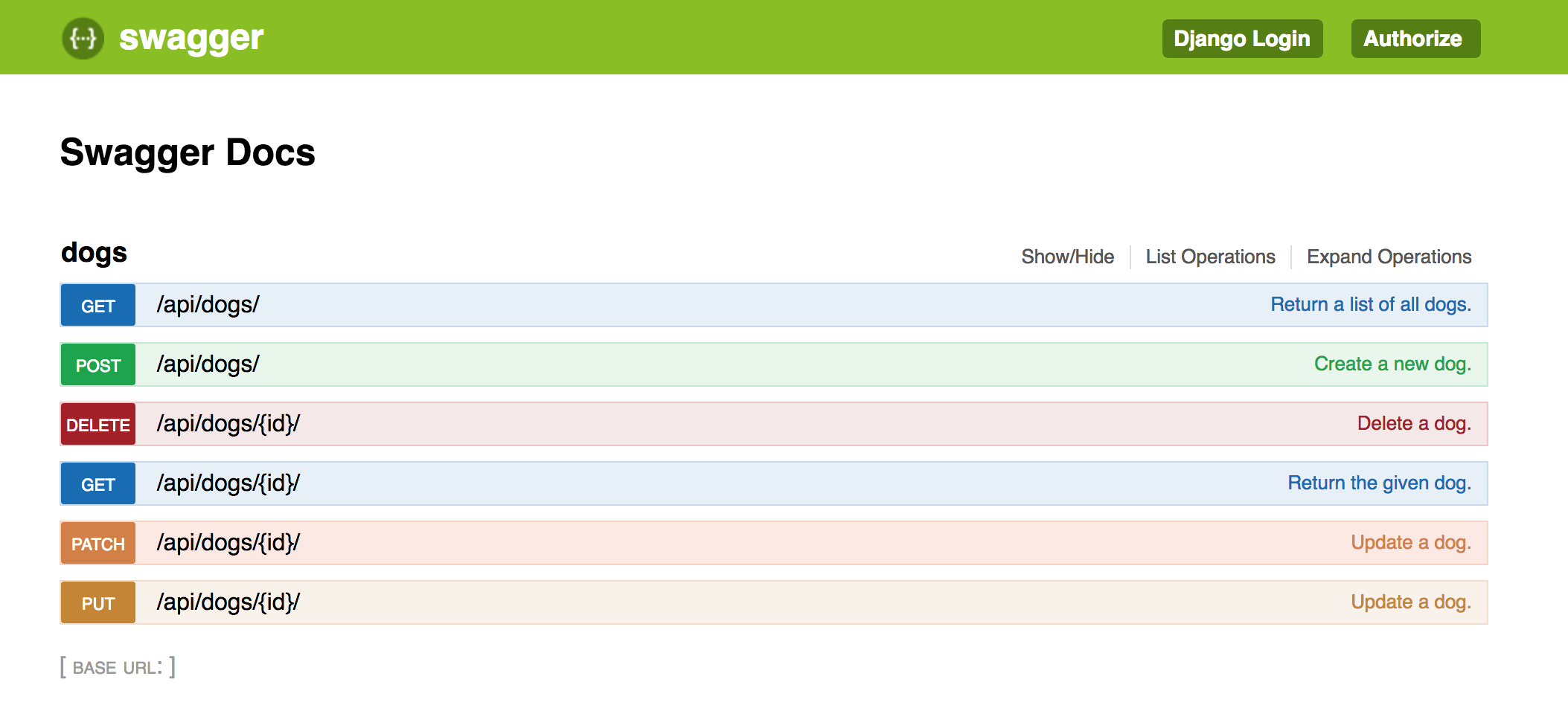
You can customize configuration for your Swagger docs by defining SWAGGER_SETTINGS
dictionary in your settings. For example, you can display request headers and list HTTP requests that allow interacting with them (only supported methods will have “Try it out!” button underneath them).
SWAGGER_SETTINGS = {
'SHOW_REQUEST_HEADERS': True,
'SUPPORTED_SUBMIT_METHODS': [
'get',
'post',
]
}
It is also possible to provide customized configuration for your authentication mechanism. For instance, if you don’t want to use Django authentication system for Swagger docs, set USE_SESSION_AUTH
to False
. This way, there won’t be a Django Login button on the top of your docs.
As you can see, it doesn’t take a lot of effort to integrate Swagger with Django REST Framework. It is straightforward and you can easily adjust settings to your preferences.